Set Interface using Java
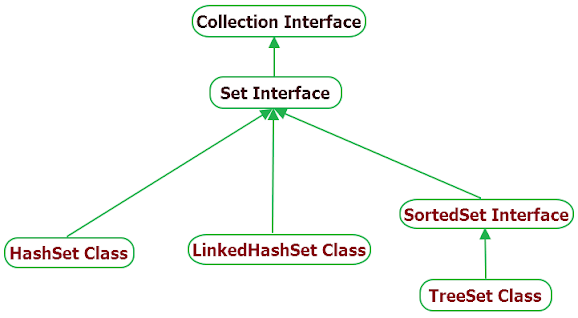
Duplicate values cannot be stored in this data structure.
1)Hash Set: Stored values in random Manner without duplication.
Set<Integer> mySet=new HashSet<>();
mySet.add(10);
mySet.add(20);
mySet.add(30);
mySet.add(10);
System.out.println(mySet.size());//out 3
System.out.println(mySet);//out [20, 10, 30]
System.out.println(mySet.contains(50));//out false
Thre are 2 method to retrieve data from Hash set
//1.using iterator
Iterator<Integer> iterator = mySet.iterator();
while(iterator.hasNext()){
System.out.println(iterator.next());
}
//2.using for each
for (Integer a:mySet) {
System.out.println(a);
}
//String
Set<String> mySet=new HashSet<>();
mySet.add("Jhone");
mySet.add("Ali");
mySet.add("Lisa");
mySet.add("Jhone");
for (String a:mySet) {
System.out.println(a);
}
Out [Ali,Jhone,Lisa]//no duplicate Jhone.
2)Link Hash Set: Store & retrieve data from insertion order without duplication.
Set<Integer> mySet = new TreeSet<>();
mySet.add(50);
mySet.add(20);
mySet.add(30);
mySet.add(10);
mySet.add(50);
*Out: [50, 20, 30, 10]
3)Tree Hash Set: Data will sore ascending order without no duplication.
Set<String> mySet2=new LinkedHashSet<>();
mySet2.add("Jhone");
mySet2.add("Lisa");
mySet2.add("Ema");
mySet2.add("Singh");
mySet2.add("Matilda");
mySet2.add("Jhone");
*Out [Ema,Jhone,Lisa,Matilda,Singh]
Objects duplication problem
When using hash set the is no any problem duplicate records will disrepair till, But when use objects problem will arise. Its there is a duplicate object,It will identify as Separate object ,then JVM as these two are separate object then it reruns duplicate records.
Solution for Hash Set & Linked Hash Set
There is two method called hashCode(), equals(Object obj) which extent from Object Class(Each class we created in java will extend from Object class automatically)
1.hashCode():
public int hashCode() {
return super.hashCode();
}
Every object,method,variable has a hash code,when the Set interface JVM identify two object with same data as deffer object .Then the duplication will happen.
instead of return super.hashCode() we can return content's hash code in that object.Then JVM identify both are same hash code.So that we want to override the hash code method in class methods
Eg- public int hashCode(){
return id.hashCode()+name.hashCode();//Return values hash code instead of object hash code
}
2.equals(Object obj):
public boolean equals(Object obj) {
return super.equals(obj);//default return
}
When the Set interface must check given data is equal or not,if it returns true then Set interface identify as duplicate data & remove it. But when we create deferent object with same content it identify as deferent object & duplication will be happen.
So that we must override that method also.
Eg:-public boolean equals(Object obj) {
return obj.hashCode()==this.hashCode();
}
Conclution :-without override both hashcode & equals method can't avoid duplication problem in object when using set interface.
Solution for Tree Hash Set
We can implements Comparable interface & override it for avoid problem.
Eq- public class Student implements Comparable {
@Override
public int compareTo(Object o) {
Student s = (Student) o;
return this.getId().compareTo(s.getId());
//return 0;
}
}
Comments
Post a Comment