Serialization Using java
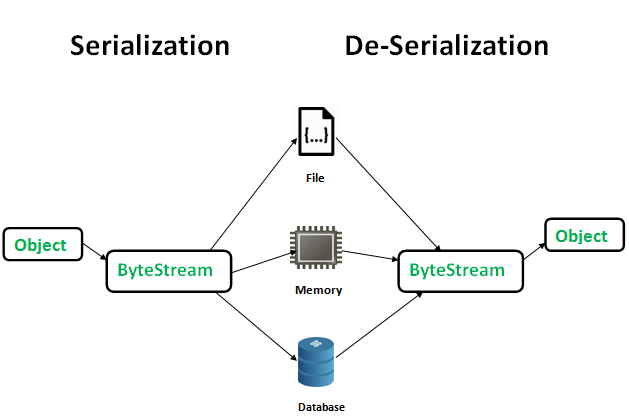
In computing, serialization is the process of translating data structures or object state into a format that can be stored or transmitted and reconstructed later is called as serialization.
Simply,Java is an object oriented programming language.Machines store files as byte stream in any file in digital world.Java have many object which include data , & it can convert to the byte stream & write to file.After write as byte stream(Called Serialization),we can send or store that data any where.
If you can convert that byte stream successfully as same as to the the previous object it called as the De-Serialization.
Importance of Serialization
- Communication: If you have two machines that are running the same code, and they need to communicate, an easy way is for one machine to build an object with information that it would like to transmit,
- Persistence: If you want to store the state of a particular operation in a database, it can be easily serialized to a byte array, and stored in the database for later retrieval.
- Deep Copy: If you need an exact replica of an Object, and don't want to go to the trouble of writing your own specialized clone() class, simply serializing the object to a byte array, and then de-serializing it to another object achieves this goal.
- Caching: Really just an application of the above, but sometimes an object takes 10 minutes to build, but would only take 10 seconds to de-serialize. So, rather than hold onto the giant object in memory, just cache it out to a file via serialization, and read it in later when it's needed.
- Cross JVM Synchronization: Serialization works across different JVMs that may be running on different architectures.(Eg:-Java Remote Method Invocation (RMI)use Serialization).
Marshaling & UN-Marshaling
In the java we can find they are synonyms for serialization & De-serialization.
but there is bit deference of that.
Serialization: only the member data within that object is written to the byte stream.
Marshalling:Object is serialized(member data is serialized) + Code base is attached.
Eg1:-There is a class called Student class & it implements from Serializable interface(This is a marking interface i.e:Marker interface in Java is interfaces with no field or methods or in simple word empty interface in java is called marker interface.)which command Student class allowed for serialization.
(a)Class
import java.io.Serializable;
public class Student implements Serializable{
private int id;
private String name;
//transient key word used for indicate the non
//serialize-variable If you add this key word do not serialize
//that variable
private transient Address name;
public Student(int id, String name){
this.id = id;
this.name = name;}
public int getId() {
return id;
}
public String getName() {
return name;
}
public void setId(int id) {
this.id = id;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "Student{" +
"id=" + id +
", name='" + name + '\'' +
'}';
}
}
(b)Serialize class objects
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
public class SerializeDemo {
public static void main(String[] args) throws IOException {
Student stu1 = new Student(1, "Jhone");
Student stu2 = new Student(2, "Ann");
//create new file in temp dir
File GradeEightFile;
GradeEightFile=newFile(System.getProperty("java.io.tmpdir")+"/GradeEight.stu");
GradeEightFile.createNewFile();
//create new FileOutputStream
FileOutputStream fileOutputStream = new FileOutputStream(GradeEightFile);
//chainning to the fileOutputStream to the object
ObjectOutputStream obis =new ObjectOutputStream(fileOutputStream);
//write the object
obis.writeObject(stu1);
obis.writeObject(stu2);
obis.close();
}
}
(c)De-serialize byte stream
Cant perform Deserialization without the original class which use for the serialization.does not run the constructor in the de-serialization(if it runs default values store & wipe data so it do not run). Serial Version UID
When the JVM compile the class compiler it gives the unique key for the any class.
We can get that using "SerialVer Student"" command in java is for find the SVUID. Serialization identify the class using that class using Serial Version UID. If it changed any class JVM identify they are deffer.So there is a changing class over time rapidly We can add that key to the class when we change class time to time.
But we cant change every data in the class
E.g:-Student: private static final long serialVersionUID = -6242024554645808205L;
we can change the class,But we cant change not relevant data type to Class
import java.io.FileInputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
public class DeserializeDemo {
public static void main(String[] args) throws IOException,ClassNotFoundException{
//pointer to the file in the temp dir.
File GradeEightFile = new File(System.getProperty("java.io.tmpdir")+"GradeEight.stu");
//check the exsistance of the file
if(!GradeEightFile.exists()){
System.err.println("File Not Found");
System.exit(1);
}
//get file input stream to object
FileInputStream fis = new FileInputStream(GradeEightFile);
//convert it the object
ObjectInputStream ois = new ObjectInputStream(fis);
//read objects
Student stu1 = (Student) ois.readObject();
System.out.println(stu1);
Student stu2 = (Student) ois.readObject();
System.out.println(stu2);
}
File GradeEightFile = new File(System.getProperty("java.io.tmpdir")+"GradeEight.stu");
//check the exsistance of the file
if(!GradeEightFile.exists()){
System.err.println("File Not Found");
System.exit(1);
}
//get file input stream to object
FileInputStream fis = new FileInputStream(GradeEightFile);
//convert it the object
ObjectInputStream ois = new ObjectInputStream(fis);
//read objects
Student stu1 = (Student) ois.readObject();
System.out.println(stu1);
Student stu2 = (Student) ois.readObject();
System.out.println(stu2);
}
}
Comments
Post a Comment